Thinkable Type — A Knowledge Graph Toolkit
ThinkableType
Interwingled Information Language
Hypertext on Hypergraphs
Multidimensional Mind Mapping
Cognitive Cartography
A DSL for Data Explorers
Strange Loop Language
Tangled Hierarchy Markup Language
Knowledge Graph Toolkit
Thinkable Type
ThinkableType is a way to represent concepts, ideas and information.
It’s great at building knowledge graphs and aims to work the way you think.
It’s simple. It’s based on a hypergraph, which doesn’t sound simple, but it is:
Ted Nelson,invented,HyperText
That’s it, a series of connected ideas. Typically separated by a
,
or ->
Tim Berners-Lee -> invented -> WWW
What matters less is the syntax, and more that there are only two things:
- Symbols
- Connections
Together these form the building blocks of ThinkableType.
A New Kind of Knowledge Graph
Current knowledge graphs limit how we think. They don’t visualize information in the way it’s actually shaped.
Mind Mapping tries to squeeze complex relationships into a 2D hierarchy—stripped of context, interconnections and intuition.
ThinkableType is not about creating a simplified view of some information in your head—it’s about getting lost in the forest, discovering deep insights, and finding your way back.
Here is Think Machine, a frontend UI for the project.
Creating a ThinkableType file
To create a ThinkableType file, just create a CSV file with some connected ideas.
Ted Nelson,invented,HyperText
Tim Berners-Lee,invented,WWW
HyperText,influenced,WWW
This file gets read, parsed and then a knowledge graph gets created with all the proper connections.
Interconnections are a core idea—called interwingle. Interwingle means knowledge is deeply interconnected and there isn’t a clean way to divide it up.
Connections, and other tools like PageRank, Text Similarity and LLMs, are all included to help you make sense of your data.
ThinkableType wants you to do the hard work of thinking—and then it will do everything it can to help you hit the high notes.
Using ThinkableType
ThinkableType is a lot of things. It’s a DSL, it’s a parser, it’s a hypergraph database, it’s a text similarity vector search engine, it’s an AI Research Copilot.
But at it’s core, it’s just a library — and getting started is easy.
Installing ThinkableType
Install from NPM:
npm install @themaximalist/thinkabletype
Load ThinkableType file
You can import an existing .csv
file
import ThinkableType from "@themaximalist/thinkabletype"
const thinkabletype = ThinkableType.parse("ancient_sumerians.csv");
// or specify a different separator
const thinkabletype = ThinkableType.parse("secret_research_project.csv", {
parse: {
delimiter: " -> "
}; })
Initialize with data
You can also initialize with an existing list of nodes and connections (called Hyperedges).
const hyperedges = [
"Plato", "student", "Socrates"],
["Aristotle", "student", "Plato"]
[;
]
const thinkabletype = new ThinkableType({ hyperedges });
Build programatically
Or you can build up a file programatically
const thinkabletype = new ThinkableType();
.add("Vannevar Bush", "author", "As We May Think");
thinkabletype.add("Ted Nelson", "invented", "HyperText");
thinkabletype.add("As We May Think", "influenced", "HyperText"); thinkabletype
You can also build up a Hyperedge one symbol at a time.
const edge = thinkabletype.add("Vannevar Bush");
.add("invented");
edge.add("Memex"); edge
Visualize ThinkableType
Connections and visualizations are a core part of ThinkableType—so Force Graph 3D is supported out of the box.
const thinkabletype = new ThinkableType({
hyperedges: [
"Hercules", "son", "Zeus"],
["Hercules", "son", "Alcmene"],
[,
];
})const data = thinkabletype.graphData(); // { nodes, links } for Force Graph 3D
In addition, an interwingle
parameter is available to
control the interconnections of the graph.
Interwingle Isolated
Isolated
displays hyperedges exactly as they’re entered,
with no interconnections.
const thinkabletype = new ThinkableType({
interwingle: ThinkableType.INTERWINGLE.ISOLATED,
hyperedges: [
"Hercules", "son", "Zeus"],
["Hercules", "son", "Alcmene"],
[,
];
})
// hyperedges are displayed exactly as entered
const data = thinkabletype.graphData();
// Hercules -> son -> Zeus
// Hercules -> son -> Alcmene
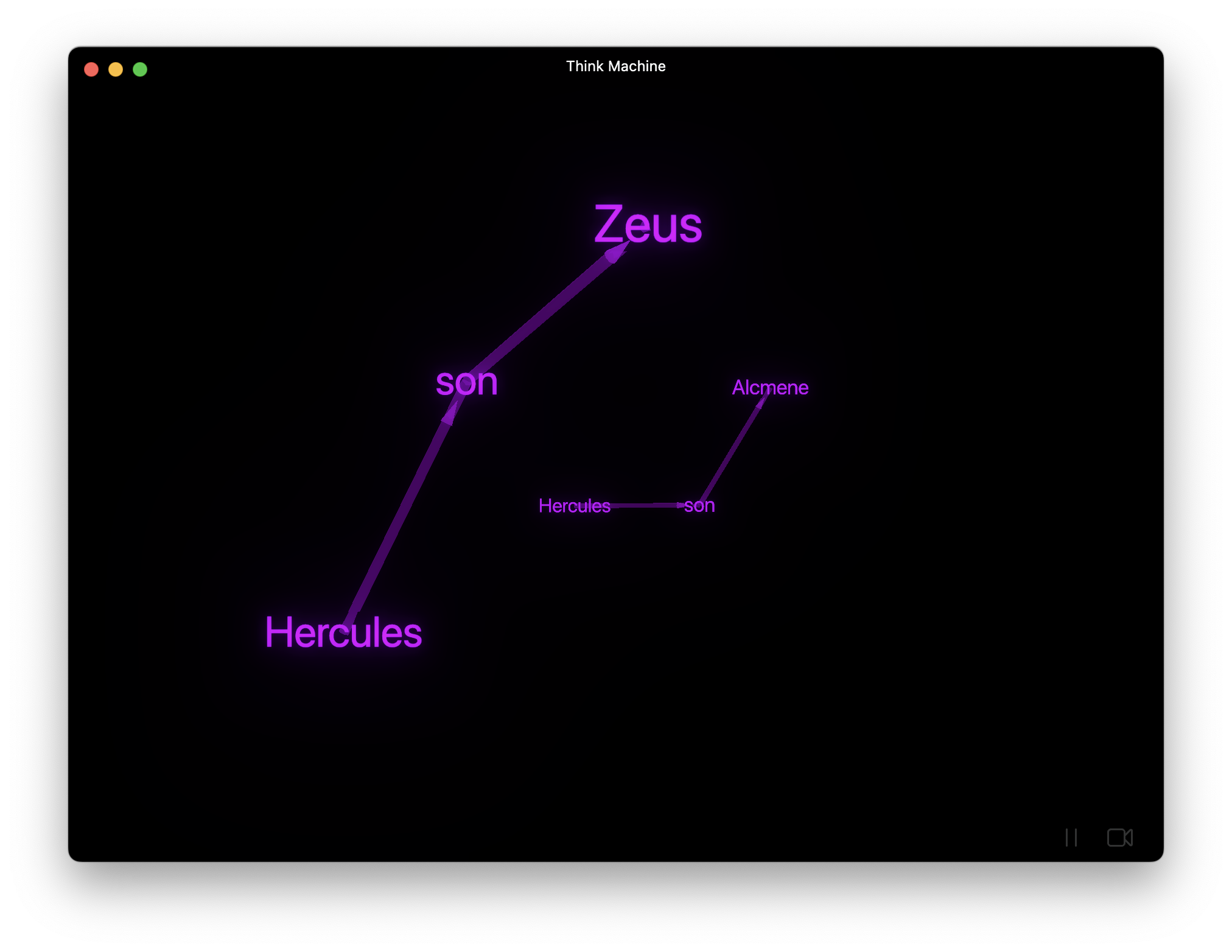
Interwingle Confluence
Confluence
connects common parents.
const thinkabletype = new ThinkableType({
interwingle: ThinkableType.INTERWINGLE.CONFLUENCE,
hyperedges: [
"Hercules", "son", "Zeus"],
["Hercules", "son", "Alcmene"],
[,
];
})
// nodes shares common ancestors
const data = thinkabletype.graphData();
//
// / Zeus
// Herculues -> son
// \ Alcmene
//
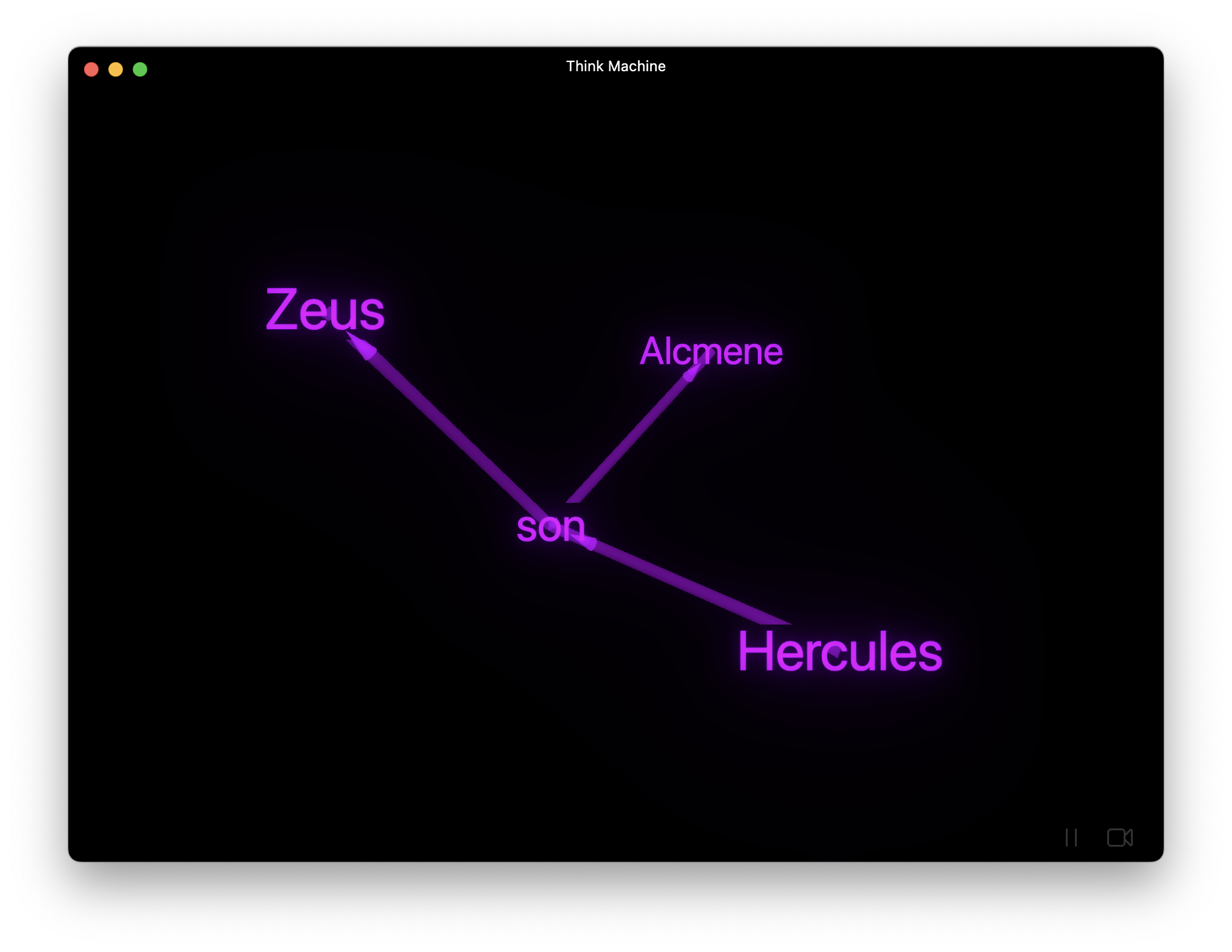
Interwingle Fusion
Fusion
connects starts, ends and direct connections.
const thinkabletype = new ThinkableType({
interwingle: ThinkableType.INTERWINGLE.FUSION,
hyperedges: [
"Plato", "student", "Socrates"],
["Aristotle", "student", "Plato"],
[,
];
})
// start and end nodes are fused together
const data = thinkabletype.graphData();
// Aristotle -> student -> Plato -> student -> Socrates
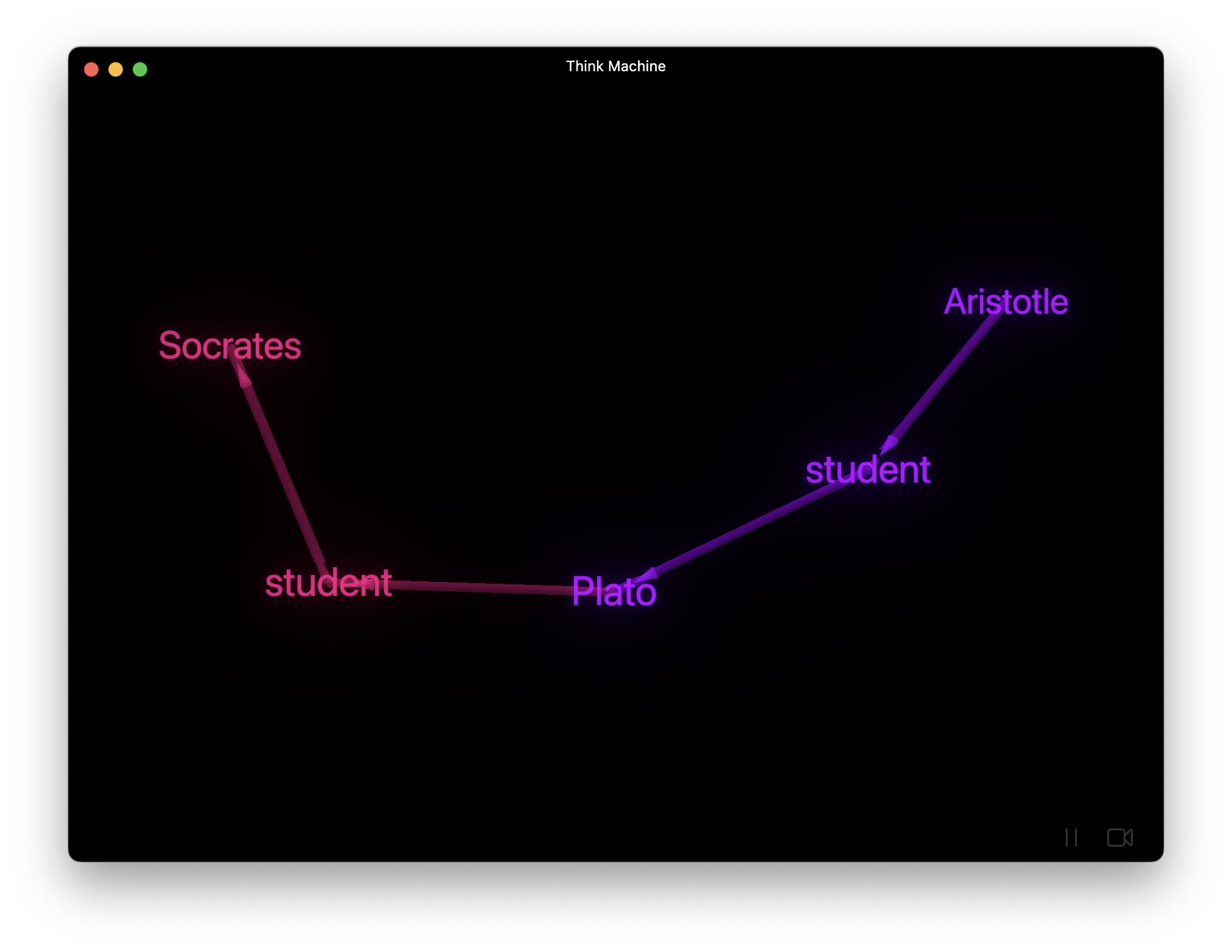
Direct Connect
When a hyperedge is two nodes long—it’s treated as a direct connection.
A,B,C
1,2,3
B,2
In this case, B,2
says link every instance of
B to every instance of 2. So the other
two edges A,B,C
and 1,2,3
are linked
together.
This is especially useful for making simpler connections than Bridge and can keep knowledge graphs structured as you intend.
Interwingle Bridge
Bridge
connects common symbols with a bridge.
const thinkabletype = new ThinkableType({
interwingle: ThinkableType.INTERWINGLE.BRIDGE,
hyperedges: [
"Vannevar Bush", "author", "As We May Think"],
["Ted Nelson", "author", "Computer Lib/Dream Machines"],
["Tim Berners-Lee", "author", "Weaving the Web"],
[,
];
})
// common symbols are connected through a bridge
const data = thinkabletype.graphData();
//
// Vannevar Bush -> author -> As We May Think
// |
// Ted Nelson -> author -> Computer Lib/Dream Machines
// |
// Tim Berners-Lee -> author -> Weaving the Web
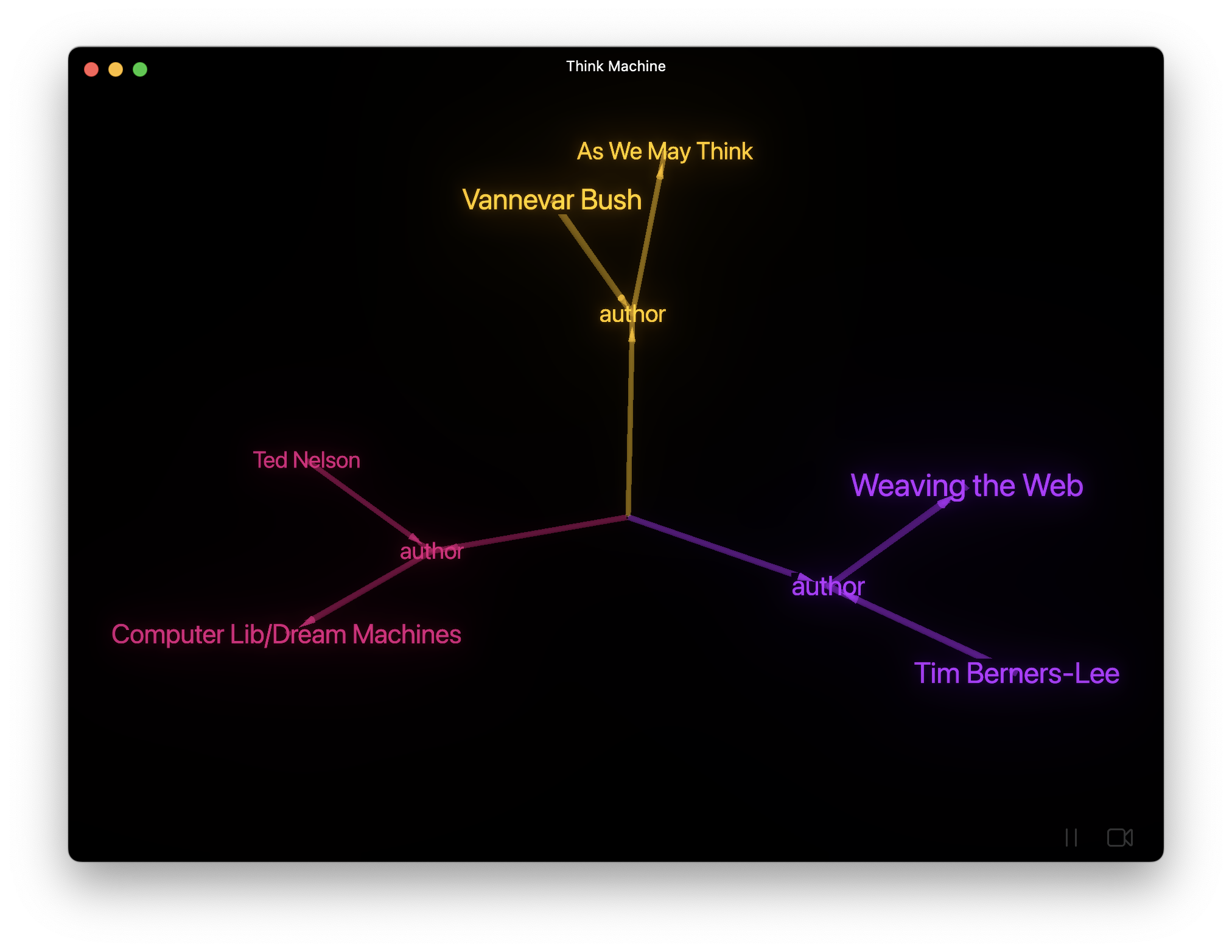
These four views give you control in how to visualize your knowledge graph and control interconnections between your data.
As you scale up the interwingle
parameter, all the
visualization layers start to combine. Using Fusion
automatically includes Confluence
. And using
Bridge
automatically includes Fusion
and
Confluence
. This lets you increase the complexity of the
knowledge graph, step-by-step.
PageRank
ThinkableType helps you find the most referenced
symbols and connections by running PageRank
on your
knowledge graph.
const thinkabletype = ThinkableType.parse(`A,B,C
A,B,D
A,B,E
A,C,Z`);
await thinkabletype.sync(); // syncs pagerank
.pageranks // { A: <num>, B: <num>, ... }
thinkabletype.pagerank("Z") // { A: <num>, C: <num>, ... } thinkabletype
Note, ThinkableType aims to work with very large knowledge
graphs, so we keep expensive operations like PageRank
explicit and in the background, controlled through
await thinkabletype.sync()
or
await thinkabletype.syncPagerank().
Embeddings and Vector Search
ThinkableType can find similar symbols and hyperedges, not only by the explicit connections, but by the text similarity.
Using Embeddings.js and VectorDB.js, you can find hidden connections in your knowledge graph.
Note, both embeddings and vector search are local by default, but you
can use embeddings from OpenAI
with a few config lines.
Find Similar Symbols
Find similar symbols in the knowledge graph, on any edge.
const thinkabletype = ThinkableType.parse(`Red,Green,Blue
White,Black,Gray`);
await thinkabletype.sync();
await thinkabletype.similarSymbols("Redish");
// [ { symbol: "Red": distance: 0.5 } ]
Find Similar Hyperedges
Find similar hyperedges based on symbols.
const thinkabletype = new ThinkableType();
const edge1 = thinkabletype.add("Red", "Green", "Blue");
const edge2 = thinkabletype.add("Red", "White", "Blue");
const edge3 = thinkabletype.add("Bob", "Sally", "Bill");
await thinkabletype.sync();
await edge1.similar(); // [ Hyperedge("Red", "White", "Blue") ]
await edge2.similar(); // [ Hyperedge("Red", "Green", "Blue") ]
Searching
Searching in ThinkableType is easy, you can search by symbol, hyperedge or a partial hyperedge.
const thinkabletype = new ThinkableType({
hyperedges: [
"Ted Nelson", "invented", "Xanadu"],
["Tim Berners-Lee", "invented", "WWW"],
["Tim Berners-Lee", "author", "Weaving the Web"],
[// ...
,
];
}).filter("invented").length; // 2
thinkabletype.filter("Tim Berners-Lee").length; // 2
thinkabletype.filter("Tim Berners-Lee", "invented").length; // 1 thinkabletype
AI Auto Suggest
ThinkableType has suggest()
built in,
which autocompletes any symbol or edge.
Using LLM.js, you can use any Large Language Model—like GPT-4, Claude, Mistral or local LLMs like Llamafile.
const options = {
llm: {
service: "openai",
model: "gpt-4-turbo-preview"
apikey: process.env.OPENAI_API_KEY
};
}
const thinkabletype = new ThinkableType(options);
const hyperedge = thinkabletype.add("Steve Jobs", "inventor");
.suggest(); // ["iPhone", "Macintosh", "iPod", ... ] thinkabletype
This makes programatically expanding knowledge graphs with LLMs incredibly easy!
AI Generate
ThinkableType also has generate()
built
in, which generates hyperedges based on your prompt. This is a great way
to expand your knowledge graph in a particular direction.
Just like with AI Auto Suggest, you can use any LLM.
const options = {
llm: {
service: "openai",
model: "gpt-4-turbo-preview",
apikey: process.env.OPENAI_API_KEY,
,
};
}
const thinkabletype = new ThinkableType(options);
const hyperedges = thinkabletype.generate("Steve Jobs");
// Steve Jobs,Apple Inc,CEO
// Steve Jobs,NeXT,Founder
// Steve Jobs,Macintosh,Personal Computers
// Steve Jobs,iPad,Tablet Computing
// ...
CLI
ThinkableType ships with a command-line interfaces for searching and generating files.
> thinkabletype epic of gilgamesh
epic of gilgamesh,sumerian literature,ancient civilization
epic of gilgamesh,gilgamesh,enkidu,immortality,friendship
sumerian literature,cuneiform writing,mesopotamia
...
By default it writes to stdout, either copy and paste to a
.csv
file or redirect the out
> thinkabletype mesopotamia > mesopotamia.csv
The Design of ThinkableType
A language for ideas needs a structureless structure. That’s exactly what Stephen Wolfram found hypergraphs to be while working on his Universal Theory of Physics project.
Ted Nelson created Zig Zag as a “hyperstructure” kit—a way to represent information in HyperText systems.
ThinkableType combines these ideas, using the hypergraph as the fundamental unit in the HyperText system.
ThinkableType is a lot closer to symbolic programming than iterative programming. In iterative programming, you describe every little step. In symbolic programming, you describe the high-level concepts. It’s top-down rather than bottom-up.
Something magical happens with symbolic programming. Like a looking glass, “the program” is a reflection of the connected symbols. It’s a lot like language. You have legalese and then you have poetry.
ThinkableType is not a programming language in and of itself—but this high-level connecting of ideas is creating a sum greater than it’s parts.
ThinkableType is open source, and the file format is incredibly simple—just CSV files.
It’s goal is to give you control over the knowledge and information in your life.
File over app is a philosophy: if you want to create digital artifacts that last, they must be files you can control, in formats that are easy to retrieve and read. Use tools that give you this freedom.
—Steph Ango (Obsidian Cofounder)
Alpha
ThinkableType is under heavy development and still subject to breaking changes.
Projects
ThinkableType is currently used in the following projects:
Author
License
MIT